Building an Investor List App with Novu and Supabase
Building an Investor List App with Novu and Supabase
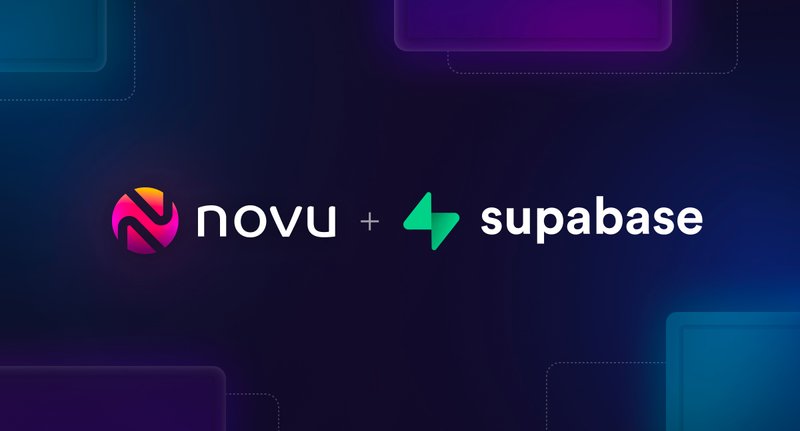
Supabase is an impressive open-source alternative to Firebase. It provides multiple tools that enable you to build comprehensive backend systems, including features for authentication, databases, storage, and real-time updates.
In this article, I’ll guide you through the process of integrating your Next.js Supabase app with Novu. This integration will allow for a seamless, quick, and stress-free notification experience.
Novu is an open-source notification infrastructure designed to assist engineering teams in creating robust product notification experiences.
We’ll create an AI investor list app. This app will enable users to add details of angel investors interested in funding AI startups at the Pre-seed, Seed, and Series A stages. It will also announce and send in-app notifications when new investors are added.
Prerequisites
Before diving into the article, make sure you have the following:
- Node.js installed on your development machine.
- A Novu account. If you don’t have one, sign up for free at the web dashboard.
- A Supabase account. If you don’t have one, sign up for free at the dashboard.
If you want to explore the code right away, you can view the completed code on GitHub.
Set up a Next.js App
To create a Next.js app, open your terminal, cd
into the directory you’d like to create the app in, and run the following command:
1npx create-next-app@latest supabase-novu
Go through the prompts:
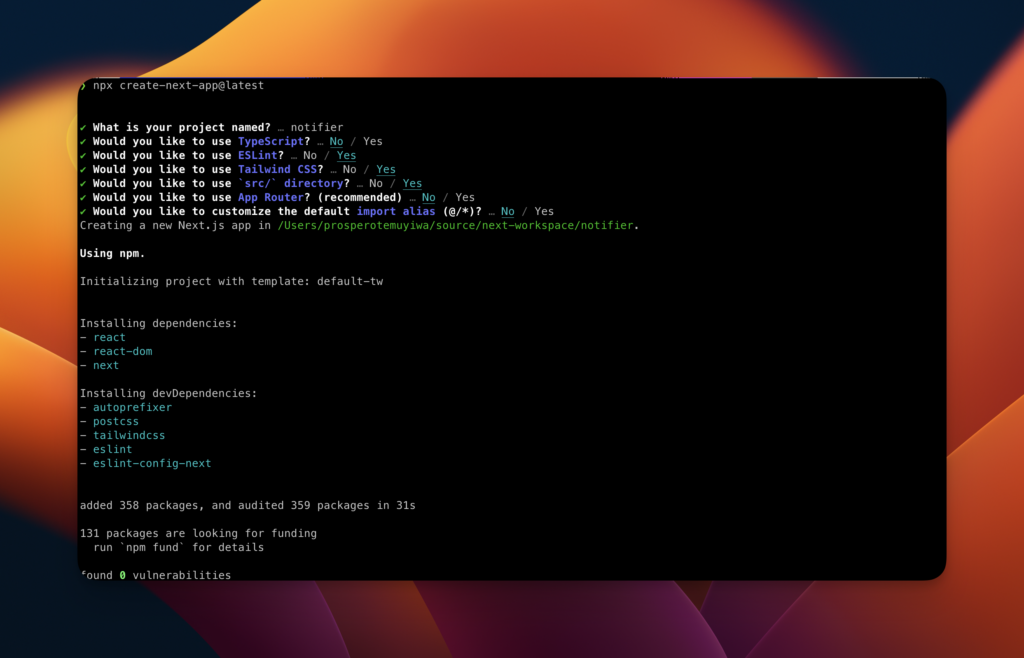
cd
into the directory, supabase-novu
and run to start the app in your browser:
1npm run dev
Set up Supabase in the App
Run the following command to add Supabase to the project:
1npm i @supabase/supabase-js
Ensure you have signed up and created a new project on Supabase. It takes a couple of minutes to have your project instance set up on the dashboard. Once done, it should look similar to the image below:
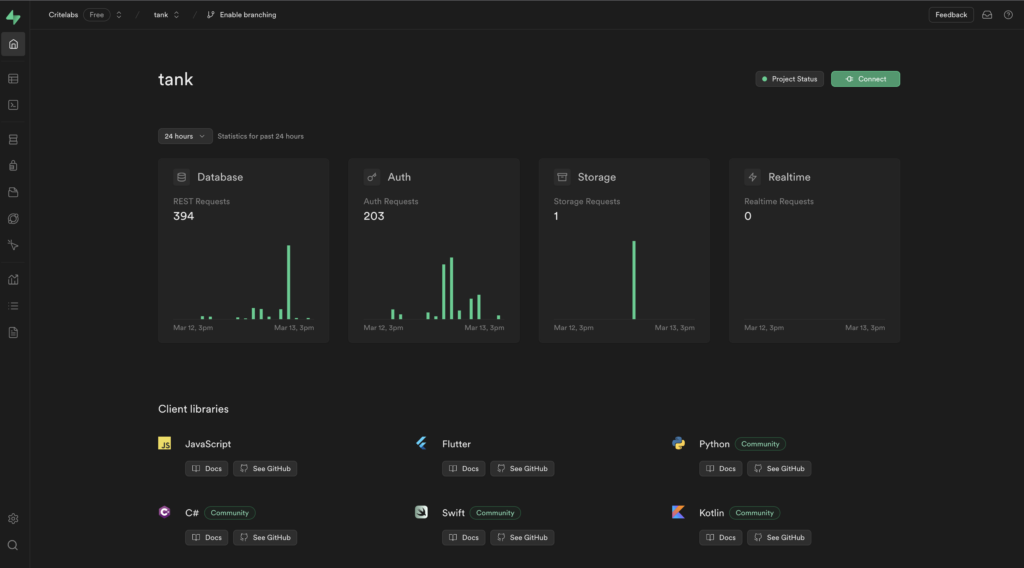
We’ll go ahead to create a database table, investors, in our newly created project. Click on the SQL Editor on the dashboard and add the following SQL query to the editor.
1CREATE TABLE investors (
2 id bigint generated by default as identity primary key,
3 email_reach text unique,
4 name text,
5 website text,
6 funding_amount text,
7 funding_type text,
8 inserted_at timestamp with time zone default timezone('utc'::text, now()) not null
9);
10
11alter table investors enable row level security;
12
13create policy "Investors are public." on investors for
14 select using (true);
It should look like so:
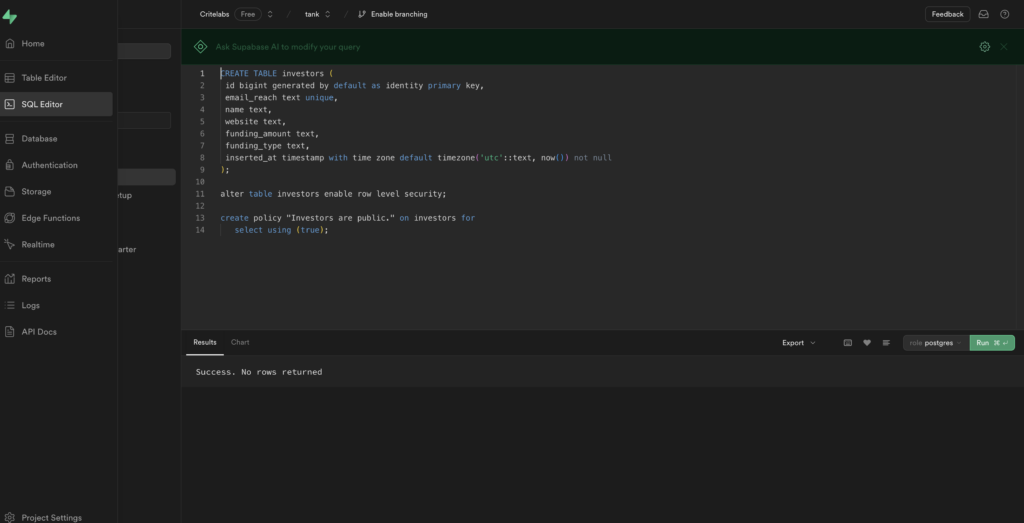
Click on “Run” to run the query, create the table and apply a Policy.
This will go ahead to create an investors
table. It also enables row level permission that allows anyone to query for all investors. In this article we are not concerned with authentication. However, this is something you should consider if you are to make it a production grade app.
You should be able to see your table created successfully!
Next, we need to connect our Next.js app with our Supabase backend.
Connect Supabase with Next.js
Create a .env.local
or .env
file in the root of the Next.js app and add the following environment variables:
1NEXT_PUBLIC_SUPABASE_URL=<insert-supabase-db-url>
2NEXT_PUBLIC_SUPABASE_ANON_KEY=<insert-anon-key>
Please replace the placeholders here with the correct values from your dashboard.
Click on the “Project Settings icon” at the bottom left and click on “API” to show you the screen below to obtain the correct values.
Create an utils
folder inside the src
directory of our Next.js project. Then go ahead to create a supabase.js
file in it. Add the code below:
1import { createClient } from "@supabase/supabase-js";
2
3const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL;
4const supabaseKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY;
5
6export const supabase = createClient(supabaseUrl, supabaseKey);
This is the Supabase client that we can use anywhere in our app going forward. The createClient
function that we imported from the supabase library makes it possible.
Next, let’s set up our UI components and wire them to the Supabase backend.
Build UI to Interact with Supabase
Open up the src/pages/index.js
file. We will modify it a lot.
Replace the content of the file with the code below:
1import Image from "next/image";
2import { Inter } from "next/font/google";
3import { supabase } from "@/utils/supabase";
4import { useEffect, useState } from "react";
5
6const inter = Inter({ subsets: ["latin"] });
7
8export default function Home() {
9 const [investors, setInvestors] = useState([]);
10 const [loading, setLoading] = useState(true);
11
12 useEffect(() => {
13 fetchAllInvestors();
14 }, []);
15
16 async function fetchAllInvestors() {
17 try {
18 setLoading(true);
19 const { data, error } = await supabase.from("investors").select("*");
20
21 if (error) throw error;
22 setInvestors(data);
23 } catch (error) {
24 alert(error.message);
25 } finally {
26 setLoading(false);
27 }
28 }
29
30 async function addInvestor(
31 email_reach,
32 name,
33 website,
34 funding_amount,
35 funding_type
36 ) {
37 try {
38 const { data, error } = await supabase
39 .from("investors")
40 .insert([
41 {
42 email_reach,
43 name,
44 website,
45 funding_amount,
46 funding_type,
47 },
48 ])
49 .single();
50 if (error) throw error;
51 alert("Investor added successfully");
52 fetchAllInvestors();
53 } catch (error) {
54 alert(error.message);
55 }
56 }
57
58 function handleSubmit(e) {
59 e.preventDefault();
60
61 const email_reach = e.target.email_reach.value;
62 const name = e.target.name.value;
63 const website = e.target.website.value;
64 const funding_amount = e.target.funding_amount.value;
65 const funding_type = e.target.funding_type.value;
66
67 addInvestor(email_reach, name, website, funding_amount, funding_type);
68 }
69
70 return (
71 <main
72 className={`flex min-h-screen flex-col items-center justify-between p-24 ${inter.className}`}
73 >
74 <div className="z-10 max-w-5xl w-full items-center justify-between font-mono text-sm lg:flex">
75 <p className="fixed left-0 top-0 flex w-full justify-center border-b border-gray-300 bg-gradient-to-b from-zinc-200 pb-6 pt-8 backdrop-blur-2xl dark:border-neutral-800 dark:bg-zinc-800/30 dark:from-inherit lg:static lg:w-auto lg:rounded-xl lg:border lg:bg-gray-200 lg:p-4 lg:dark:bg-zinc-800/30">
76 NOVU SUPABASE DASHBOARD
77 </p>
78 <div className="fixed bottom-0 left-0 flex h-48 w-full items-end justify-center bg-gradient-to-t from-white via-white dark:from-black dark:via-black lg:static lg:h-auto lg:w-auto lg:bg-none">
79 <a
80 className="pointer-events-none flex place-items-center gap-2 p-8 lg:pointer-events-auto lg:p-0"
81 href="<https://vercel.com?utm_source=create-next-app&utm_medium=default-template-tw&utm_campaign=create-next-app>"
82 target="_blank"
83 rel="noopener noreferrer"
84 >
85 By{" "}
86 <Image
87 src="/vercel.svg"
88 alt="Vercel Logo"
89 className="dark:invert"
90 width={100}
91 height={24}
92 priority
93 />
94 </a>
95 </div>
96 </div>
97
98 <div class="grid grid-cols-2">
99 <div className="mt-1 flex justify-center">
100 <form onSubmit={handleSubmit}>
101 <div>
102 <label className="p-3 block">Name:</label>
103 <input
104 className="text-black p-2"
105 type="text"
106 name="name"
107 required
108 placeholder="Enter name"
109 />
110 </div>
111 <div>
112 <label className="p-3 block">Email Reach:</label>
113 <input
114 className="text-black p-2"
115 type="text"
116 name="email_reach"
117 required
118 placeholder="Enter investor email"
119 />
120 </div>
121 <div className="mt-5">
122 <label className="p-2 block">Website:</label>
123 <input
124 className="text-black p-2"
125 type="text"
126 name="website"
127 required
128 placeholder="Enter website"
129 />
130 </div>
131 <div className="mt-5">
132 <label className="p-2 block">Funding Amount (Up to X USD):</label>
133 <input
134 className="text-black p-2"
135 type="text"
136 name="funding_amount"
137 required
138 placeholder="Enter funding amount"
139 />
140 </div>
141 <div className="mt-5">
142 <label className="p-2 block">Funding Type:</label>
143 <input
144 className="text-black p-2"
145 type="text"
146 name="funding_type"
147 required
148 placeholder="Enter type of Funding"
149 />
150 </div>
151
152 <button
153 type="submit"
154 className="bg-blue-600 p-2 rounded-md mt-5 px-12"
155 >
156 Submit Investor Details
157 </button>
158 </form>
159 </div>
160
161 <div className="mt-1 flex justify-center">
162 {investors?.length === 0 ? (
163 <div>
164 <p>There are no investors yet</p>
165 </div>
166 ) : (
167 <div>
168 <p className="mb-5">Here are the investors available: </p>
169 <table>
170 <thead>
171 <tr>
172 <th>Name </th>
173 <th>Email Reach</th>
174 <th>Website</th>
175 <th className="p-3">Funding Amt</th>
176 <th>Funding Type </th>
177 </tr>
178 </thead>
179 <tbody className="">
180 {investors?.map((item) => (
181 <tr key={item.id}>
182 <td>{item.name}</td>
183 <td>{item.email_reach}</td>
184 <td>{item.website}</td>
185 <td>{item.funding_amount}</td>
186 <td>{item.funding_type}</td>
187 </tr>
188 ))}
189 </tbody>
190 </table>
191 </div>
192 )}
193 </div>
194 </div>
195
196 <div className="mb-32 grid text-center lg:max-w-5xl lg:w-full lg:mb-0 lg:grid-cols-4 lg:text-left">
197 <a
198 href="<https://nextjs.org/docs?utm_source=create-next-app&utm_medium=default-template-tw&utm_campaign=create-next-app>"
199 className="group rounded-lg border border-transparent px-5 py-4 transition-colors hover:border-gray-300 hover:bg-gray-100 hover:dark:border-neutral-700 hover:dark:bg-neutral-800/30"
200 target="_blank"
201 rel="noopener noreferrer"
202 >
203 <h2 className={`mb-3 text-2xl font-semibold`}>
204 Docs{" "}
205 <span className="inline-block transition-transform group-hover:translate-x-1 motion-reduce:transform-none">
206 ->
207 </span>
208 </h2>
209 <p className={`m-0 max-w-[30ch] text-sm opacity-50`}>
210 Find in-depth information about Next.js features and API.
211 </p>
212 </a>
213
214 <a
215 href="<https://nextjs.org/learn?utm_source=create-next-app&utm_medium=default-template-tw&utm_campaign=create-next-app>"
216 className="group rounded-lg border border-transparent px-5 py-4 transition-colors hover:border-gray-300 hover:bg-gray-100 hover:dark:border-neutral-700 hover:dark:bg-neutral-800/30"
217 target="_blank"
218 rel="noopener noreferrer"
219 >
220 <h2 className={`mb-3 text-2xl font-semibold`}>
221 Learn{" "}
222 <span className="inline-block transition-transform group-hover:translate-x-1 motion-reduce:transform-none">
223 ->
224 </span>
225 </h2>
226 <p className={`m-0 max-w-[30ch] text-sm opacity-50`}>
227 Learn about Next.js in an interactive course with quizzes!
228 </p>
229 </a>
230
231 <a
232 href="<https://vercel.com/templates?framework=next.js&utm_source=create-next-app&utm_medium=default-template-tw&utm_campaign=create-next-app>"
233 className="group rounded-lg border border-transparent px-5 py-4 transition-colors hover:border-gray-300 hover:bg-gray-100 hover:dark:border-neutral-700 hover:dark:bg-neutral-800/30"
234 target="_blank"
235 rel="noopener noreferrer"
236 >
237 <h2 className={`mb-3 text-2xl font-semibold`}>
238 Templates{" "}
239 <span className="inline-block transition-transform group-hover:translate-x-1 motion-reduce:transform-none">
240 ->
241 </span>
242 </h2>
243 <p className={`m-0 max-w-[30ch] text-sm opacity-50`}>
244 Discover and deploy boilerplate example Next.js projects.
245 </p>
246 </a>
247
248 <a
249 href="<https://vercel.com/new?utm_source=create-next-app&utm_medium=default-template-tw&utm_campaign=create-next-app>"
250 className="group rounded-lg border border-transparent px-5 py-4 transition-colors hover:border-gray-300 hover:bg-gray-100 hover:dark:border-neutral-700 hover:dark:bg-neutral-800/30"
251 target="_blank"
252 rel="noopener noreferrer"
253 >
254 <h2 className={`mb-3 text-2xl font-semibold`}>
255 Deploy{" "}
256 <span className="inline-block transition-transform group-hover:translate-x-1 motion-reduce:transform-none">
257 ->
258 </span>
259 </h2>
260 <p className={`m-0 max-w-[30ch] text-sm opacity-50 text-balance`}>
261 Instantly deploy your Next.js site to a shareable URL with Vercel.
262 </p>
263 </a>
264 </div>
265 </main>
266 );
267}
Let’s go over some sections of this code block:
1function handleSubmit(e) {
2 e.preventDefault();
3
4 const email_reach = e.target.email_reach.value;
5 const name = e.target.name.value;
6 const website = e.target.website.value;
7 const funding_amount = e.target.funding_amount.value;
8 const funding_type = e.target.funding_type.value;
9
10 addInvestor(email_reach, name, website, funding_amount, funding_type);
11 }
This function handles the submission of the form. It grabs the value from the form fields and passes it to the `addInvestor` function.
1async function addInvestor(
2 email_reach,
3 name,
4 website,
5 funding_amount,
6 funding_type
7 ) {
8 try {
9 const { data, error } = await supabase
10 .from("investors")
11 .insert([
12 {
13 email_reach,
14 name,
15 website,
16 funding_amount,
17 funding_type,
18 },
19 ])
20 .single();
21 if (error) throw error;
22 alert("Investor added successfully");
23 fetchAllInvestors();
24 } catch (error) {
25 alert(error.message);
26 }
27 }
28
The addInvestor
function takes in the right arguments and makes a query to supabase to insert the values into the investors
table.
This function also calls the fetchAllInvestors()
function that retrieves all data from the investors
table.
1async function fetchAllInvestors() {
2 try {
3 setLoading(true);
4 const { data, error } = await supabase.from("investors").select("*");
5
6 if (error) throw error;
7 setInvestors(data);
8 } catch (error) {
9 alert(error.message);
10 } finally {
11 setLoading(false);
12 }
13 }
14
As you can see from the code above, the fetchAllInvestors()
function makes a SELECT query to the investors
table to return and all the investors to the frontend.
1const [investors, setInvestors] = useState([]);
2const [loading, setLoading] = useState(true);
3
4useEffect(() => {
5 fetchAllInvestors();
6}, []);
When the page loads up, it calls the fetchAllInvestors()
function by default.
Run your app with npm run dev
. It should look like this:
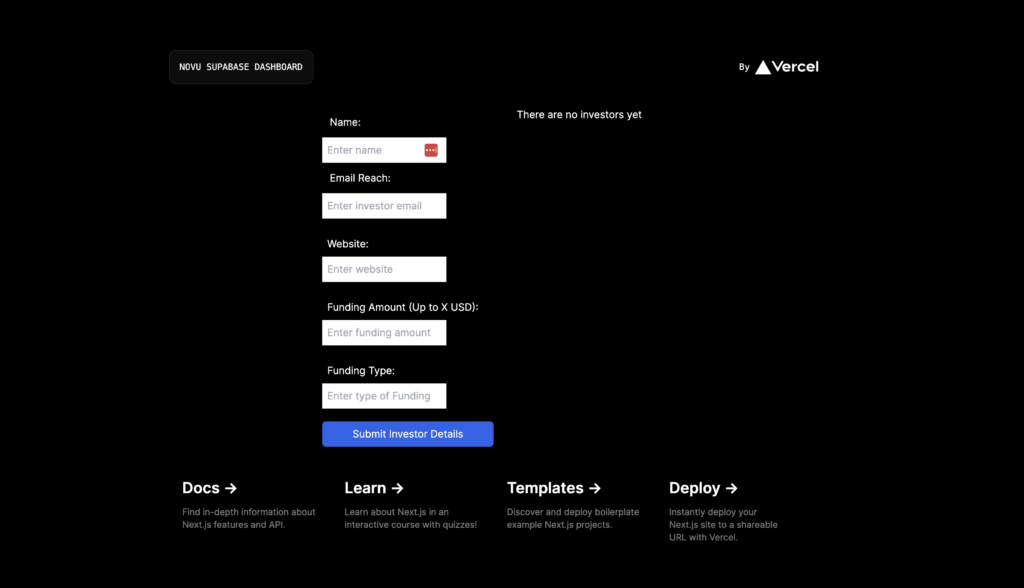
Add some investor details and submit. Your app should look like this now:
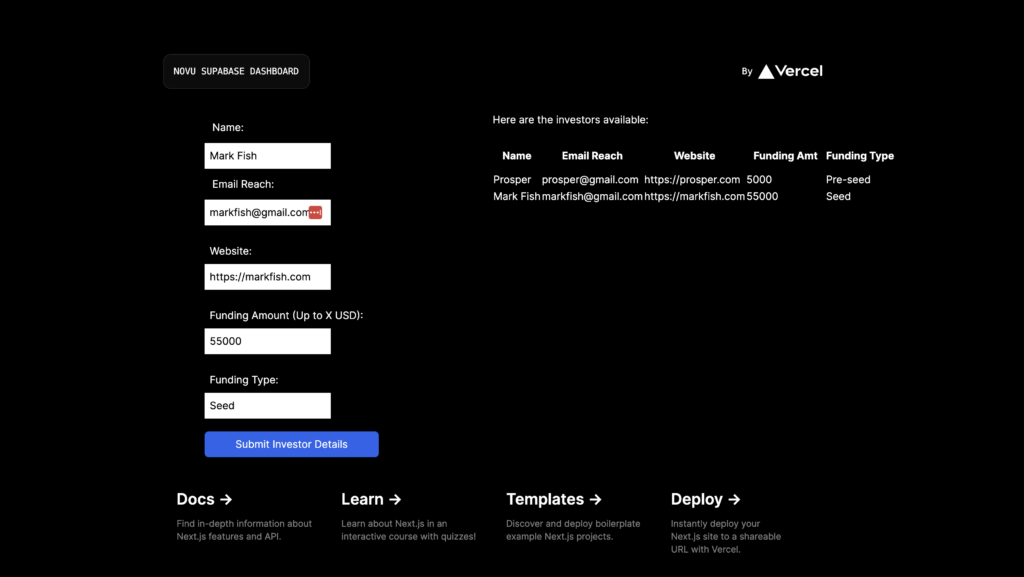
As we add items, they are saved to the Supabase database and displayed on the screen.
Inspect the table on Supabase. The data should appear as follows:
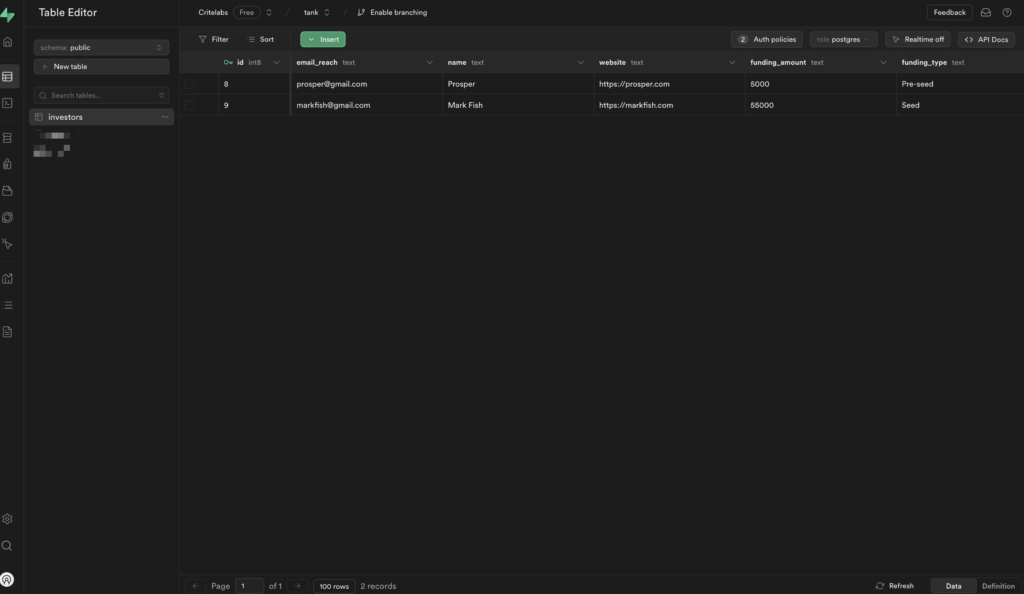
Next, we’ll set up notifications in the app. Our goal is to alert everyone when a new investor is added to the database. This is where Novu comes into play!
Set up Novu in the App
Run the following command to install the Novu node SDK:
1npm install @novu/node
2
Run the following command to install the Novu Notification Center package:
1npm install @novu/notification-center
The Novu Notification Center package provides a React component library that adds a notification center to your React app. The package is also available for non-React apps.
Before we can start sending and receiving notifications, we need to set up a few things:
- Create a workflow for sending notifications,
- Create a subscriber – recipient of notifications.
Create a Novu Workflow
A workflow is a blueprint for notifications. It includes the following:
- Workflow name and Identifier
- Channels: – Email, SMS, Chat, In-App and Push
Follow the steps below to create a workflow:
- Click Workflow on the left sidebar of your Novu dashboard.
- Click the Add a Workflow button on the top left. You can select a Blank workflow or use one of the existing templates.
- The name of the new workflow is currently “Untitled”. Rename it to
notify users of new investors
. - Select In-App as the channel you want to add. !https://mintlify.s3-us-west-1.amazonaws.com/novu/images/in-app-react.png
- Click on the recently added “In-App” channel and add the following text to it. Once you’re done, click “Update” to save your configuration.
The {{name}},{{funding_amount}}
and {{email}}
are custom variables. This means that we can pass them to our payload before we trigger a notification. You’ll see this when we create the API route to send notifications.
Create a subscriber
If you click “Subscriber” on the left sidebar of the Novu dashboard, you’ll see the subscriber list. As a first time Novu user, it will be an empty list. Subscribers are your app users.
Open your terminal and run the following script to create a subscriber:
1curl --location '<https://api.novu.co/v1/subscribers>' \\
2 --header 'Content-Type: application/json' \\
3 --header 'Accept: application/json' \\
4 --header 'Authorization: ApiKey <NOVU_API_KEY>' \\
5 --data-raw '{
6 "firstName": "John",
7 "lastName": "Doe",
8 "email": "[email protected]",
9 "phone": "+1234567890"
10 }'
Note: You can get your NOVU API Key from the settings section of your Novu dashboard.
Refresh the Subscribers page on your Novu dashboard. You should see the recently added subscriber now! You can also add a subscriber to Novu by running this API endpoint.
The best option to add a subscriber is via code in your backend. With Node.js code, you can run the following code to create a subscriber:
1import { Novu } from "@novu/node";
2
3// Insert your Novu API Key here
4const novu = new Novu("<NOVU_API_KEY>");
5
6// Create a subscriber on Novu
7await novu.subscribers.identify("132", {
8 email: "[email protected]",
9 firstName: "John",
10 lastName: "Doe",
11 phone: "+13603963366",
12});
Set up Novu Notification Center in the App
Head over to scr/pages/index.js
. We’ll modify this page again to include the following:
- Import and display the Novu Notification Center.
- A function to trigger the notification when a new investor is added.
Import the Notification Center components from the Novu notification center package like so:
1...
2import {
3 NovuProvider,
4 PopoverNotificationCenter,
5 NotificationBell,
6} from "@novu/notification-center";
Display the notification center by adding the imported components like so:
1...
2return (
3 <main
4 className={`flex min-h-screen flex-col items-center justify-between p-24 ${inter.className}`}
5 >
6 <div className="z-10 max-w-5xl w-full items-center justify-between font-mono text-sm lg:flex">
7 <p className="fixed left-0 top-0 flex w-full justify-center border-b border-gray-300 bg-gradient-to-b from-zinc-200 pb-6 pt-8 backdrop-blur-2xl dark:border-neutral-800 dark:bg-zinc-800/30 dark:from-inherit lg:static lg:w-auto lg:rounded-xl lg:border lg:bg-gray-200 lg:p-4 lg:dark:bg-zinc-800/30">
8 NOVU SUPABASE DASHBOARD
9 </p>
10 <div className="fixed bottom-0 left-0 flex h-48 w-full items-end justify-center bg-gradient-to-t from-white via-white dark:from-black dark:via-black lg:static lg:h-auto lg:w-auto lg:bg-none">
11 <NovuProvider
12 subscriberId={process.env.NEXT_PUBLIC_SUBSCRIBER_ID}
13 applicationIdentifier={process.env.NEXT_PUBLIC_NOVU_APP_ID}
14 >
15 <PopoverNotificationCenter>
16 {({ unseenCount }) => (
17 <NotificationBell unseenCount={unseenCount} />
18 )}
19 </PopoverNotificationCenter>
20 </NovuProvider>
21 </div>
22 </div>
23
24 ...
25 <div className="grid grid-cols-2">
Now, your app should display a notification bell. When this bell is clicked, a notification user interface will pop up.
Note: The NovuProvider root Component ships with many props that can be used to customize the Notification Center to your taste. The floating popover component that appears when clicking on the NotificationBell button. It renders the NotificationCenter component inside its content.
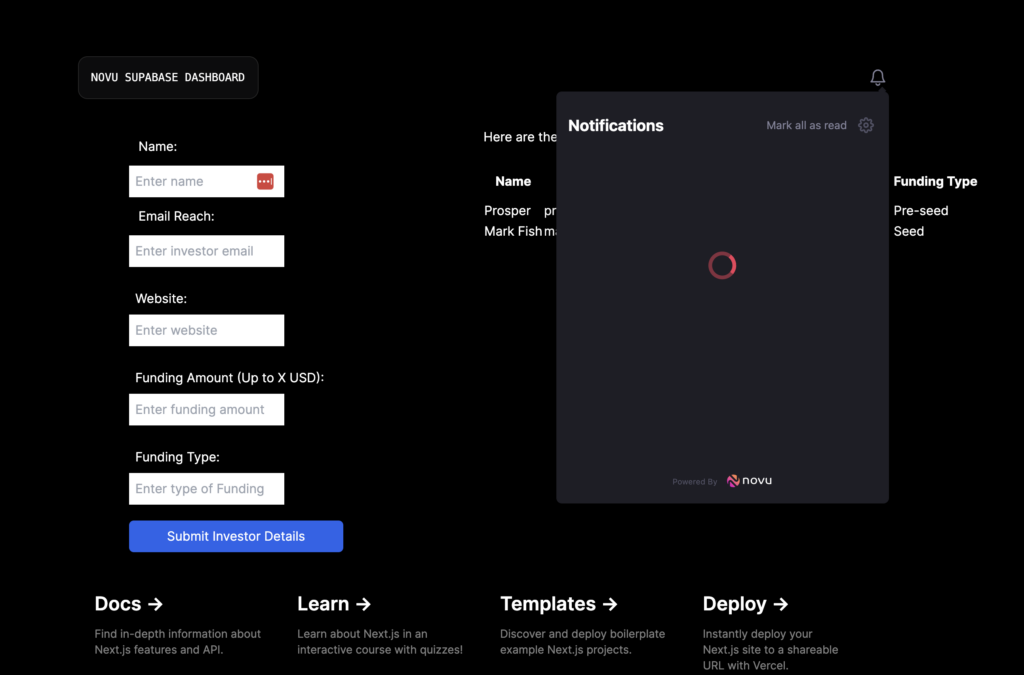
Add the following Novu env variables to your .env.local
file:
1NEXT_PUBLIC_SUBSCRIBER_ID=
2NEXT_PUBLIC_NOVU_APP_ID=
3NEXT_PUBLIC_NOVU_API_KEY=
The Novu API Key and APP ID can be found in the Settings section of your Novu dashboard.
The Subscriber ID too is on your dashboard. Earlier in this article, we created a subscriber. We need that ID now.
Note: Adding the Subscriber ID to the env file is only for the purpose of this article. Your subscriber ID is the user ID obtained from an authenticated user. When a user logs in, their ID should be the value passed to the subscriberId property of the NovuProvider component.
Develop a Simple API to Trigger Notifications
Create a send-notification.js
file in the src/pages/api
directory. Add the code below to it:
1import { Novu } from "@novu/node";
2
3const novu = new Novu(process.env.NEXT_PUBLIC_NOVU_API_KEY);
4
5export const workflowTriggerID = "notify-users-of-new-investors";
6
7export default async function handler(req, res) {
8 const { email, name, amount } = JSON.parse(req.body);
9
10 await novu.trigger(workflowTriggerID, {
11 to: {
12 subscriberId: process.env.NEXT_PUBLIC_SUBSCRIBER_ID,
13 },
14 payload: {
15 name: name,
16 funding_amount: amount,
17 email: email,
18 },
19 });
20
21 return res.json({ finish: true });
22}
23
The workflowTriggerID
value is obtained from the workflow dashboard. Earlier, when we set up a workflow titled notify users of new investors
, Novu created a slug from this title to serve as the trigger ID.
You can see it here:
The block of code below triggers the notification via the Novu SDK:
- Accepts the workflow trigger ID to determine which workflow to trigger.
- Accepts the subscriber ID value to identify the notification recipient.
- Accepts a payload object that represents the parameters to inject into the workflow variables.
1await novu.trigger(workflowTriggerID, {
2 to: {
3 subscriberId: process.env.NEXT_PUBLIC_SUBSCRIBER_ID,
4 },
5 payload: {
6 name: name,
7 funding_amount: amount,
8 email: email,
9 },
10 });
Next, we need to set up one more thing on the frontend before we test the notification experience in our app.
Add a Notification Function to Index.js
Create a function inside the Home()
function to call our recently created API like so:
1async function triggerNotification(email_reach, name, funding_amount) {
2 await fetch("/api/send-notification", {
3 method: "POST",
4 body: JSON.stringify({
5 email: email_reach,
6 name: name,
7 amount: funding_amount,
8 }),
9 });
10 }
Call the triggerNotification
function just after the addInvestor
function within the handleSubmit
function.
Your handleSubmit
function should now appear as follows:
1function handleSubmit(e) {
2 e.preventDefault();
3
4 const email_reach = e.target.email_reach.value;
5 const name = e.target.name.value;
6 const website = e.target.website.value;
7 const funding_amount = e.target.funding_amount.value;
8 const funding_type = e.target.funding_type.value;
9
10 addInvestor(email_reach, name, website, funding_amount, funding_type);
11 triggerNotification(email_reach, name, funding_amount);
12 }
Run your app again and submit a new investor detail. You should get an instant In-App notification with the details of the new investor.
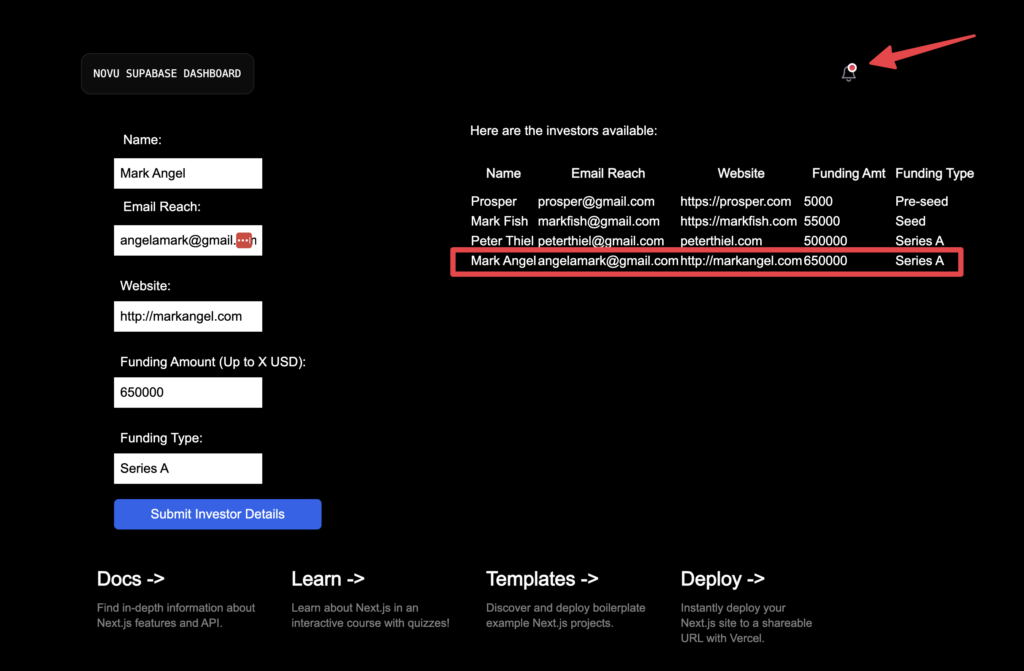
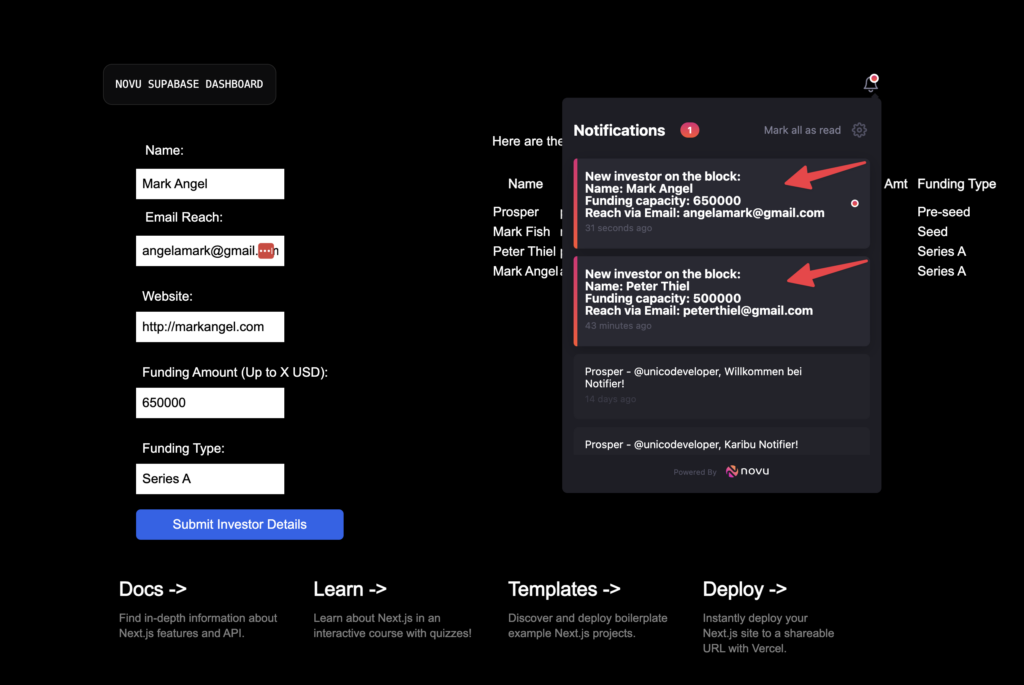
A Step Further – Notify All Investors
We currently have a database containing investor emails. This is valuable data. Let’s consider adding a feature to email these investors about new investment rounds from startups seeking funding.
We will not add a UI for the save of brevity. You can add a UI as an improvement and challenge.
Create a new file, email-investors.js
within the pages/api
directory.
Add the code below to it:
1import { Novu } from "@novu/node";
2import { supabase } from "@/utils/supabase";
3
4const novu = new Novu(process.env.NEXT_PUBLIC_NOVU_API_KEY);
5
6export const workflowTriggerID = "new-opportunities";
7
8export default async function handler(req, res) {
9 /**
10 * Grab all investors
11 */
12 const { data, error } = await supabase.from("investors").select("*");
13
14 /**
15 * Map into a new array of subscriber data
16 */
17 const subscriberData = data.map((item) => {
18 return {
19 subscriberId: item.id.toString(),
20 email: item.email_reach,
21 };
22 });
23
24 const extractIDs = (data) => {
25 return data.map((item) => item.subscriberId);
26 };
27
28 const subscriberIDs = extractIDs(subscriberData);
29
30 /**
31 * Bulk create subscribers on Novu
32 */
33 await novu.subscribers.bulkCreate(subscriberData);
34
35 /**
36 * Create a Topic on Novu
37 */
38 await novu.topics.create({
39 key: "all-investors",
40 name: "List of all investors on our platform",
41 });
42
43 /**
44 * Assign subscribers to Topic
45 */
46 const topicKey = "all-investors";
47
48 /**
49 * Add all investors to the Topic
50 */
51 await novu.topics.addSubscribers(topicKey, {
52 subscribers: subscriberIDs,
53 });
54
55 /**
56 * Trigger workflow to the Topic
57 */
58 await novu.trigger(workflowTriggerID, {
59 to: [{ type: "Topic", topicKey: topicKey }],
60 });
61
62 return res.json({ finish: true });
63}
64
There are a series of events happening in this file. I’ll explain the steps below:
- Fetch all investors data from Supabase
- Return an array of objects of the supabase data with “subscriberId” and “email”.
- Create all the investors as subscribers on Novu. Thankfully Novu has a `bulkCreate” function to create hundreds of subscribers at once instead of doing a complex loop yourself.
- Create a topic on Novu. Topics offers a great way of sending notifications to multiple subscribers at once. In this case, it’s perfect for us. Here, we created a “all-investors” topic and then proceeded to add all the investors as subscribers to the topic.
- The last step is what triggers the notification to all the investors. This is a simple syntax that triggers notifications to a topic. This means every subscriber belonging to that topic will receive a notification!
This block of code is where I set the trigger ID of the new Email workflow i created.
1export const workflowTriggerID = "new-opportunities";
2
If you haven’t, go ahead and create an email workflow on Novu. Name the workflow and use the corresponding trigger ID like I did.
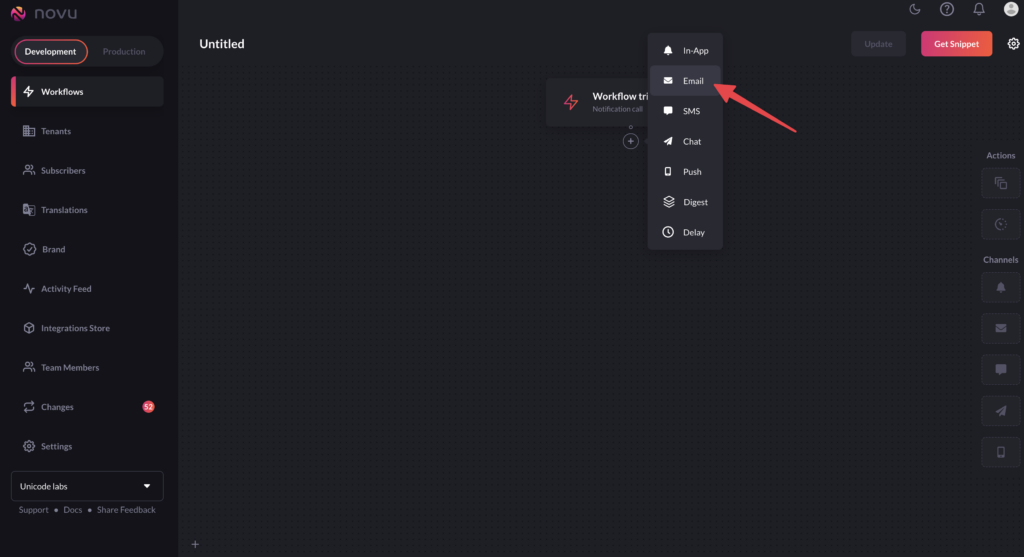
Add content to the email workflow and save.
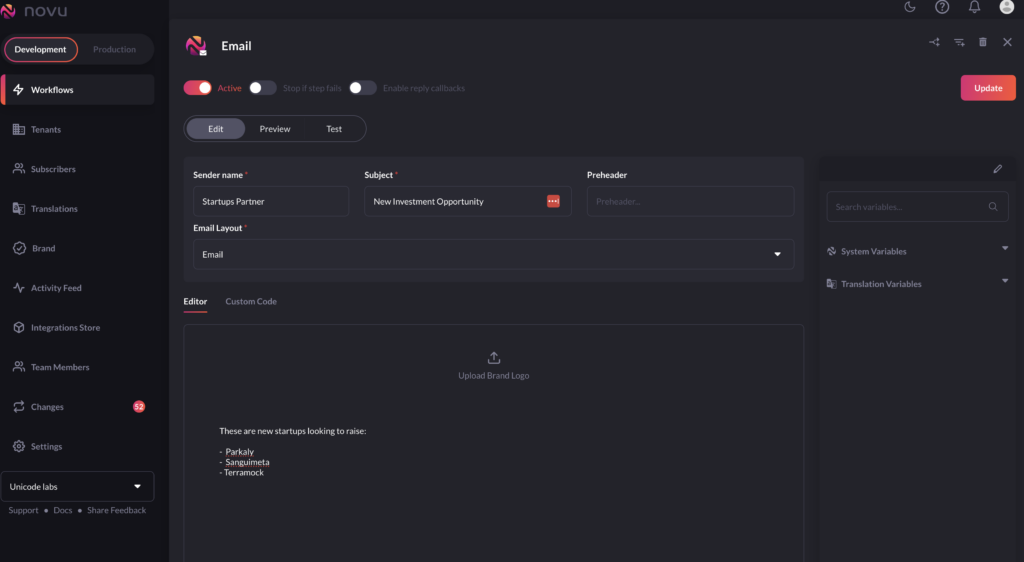
Note: At this stage, it’s crucial to ensure that an email provider has been selected from the Integrations Store. This allows Novu to identify the email provider to use for delivering the email.
Run the API:
-> /api/email-investors
.
The investors should get an email like this:
Conclusion
The combination of two robust open-source tools, Novu and Supabase, enables the rapid development of apps with a rich notification experience.
I hope you enjoyed building this simple investor list app as much as I did. Novu offers different channels such as Email, SMS, and Push, gives you a detailed activity feed to help debug your notifications and much more!
Do you have an idea or an app you’d like to build with Novu and Supabase? We are excited to see the incredible apps you’ll create. Don’t hesitate to ask any questions or request support. You can find us on Discord and Twitter. Please feel free to reach out.