How to send an SMS using Twilio
Communicating with users from a software application is a common feature in modern software development. Whether you provide an OTP to users before they can make transactions, send an email verification link before creating an account, mail newsletters, or send SMS notifications, you need an efficient tool that enables you to communicate with your users effectively. These are the tasks Twilio performs perfectly.
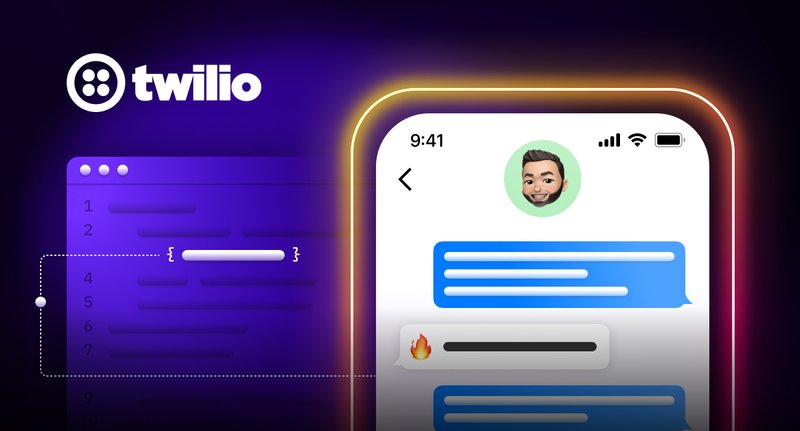
Twilio is an efficient communication tool that offers a great user experience that enables you to set up, manage, and track every communication with your users. It provides a robust API that allows you to add various forms of communication such as video chat functionality, interactive live video and audio streaming experience, phone calls, SMS, WhatsApp, emails, and chatbots to your software applications.
In addition to its well-crafted documentation, Twilio has a large community of users who create tutorials and organize events about numerous problems they have solved using Twilio and how it has contributed to the success of their businesses.
In this article, you will learn how to send messages to users via Twilio by building a Coupon Generator in Node.js. The Coupon Generator generates a random set of strings, collects the user’s phone number, and sends the coupon to the user via the phone number.
Before we go further, let’s learn more about a few reasons why you should choose Twilio.
Why choose Twilio?
In this section, you will learn more about why you should choose Twilio when adding a means of communication to your software applications.
Great user experience
Twilio has a great user experience. After creating an account, it provides a monitor tab on your dashboard where you can view insights into every activity you performed. Twilio’s website is also easy to navigate. Despite providing quite a large number of features, it ensures that you find information about the tool you are interested in right away.
A large community of users
Twilio has a large community of users, including developers and businesses. It helps organizations such as Stripe, Lyft, Airbnb, Vacasa, and lots more to provide excellent customer service to their users. Twilio also has an active developer community that organizes workshops and creates tutorials about various problems you can solve using Twilio.
Excellent documentation
Twilio has excellent documentation, making it easy for new and existing users to navigate and learn about its features quickly. The documentation explains every tool you need and provides code samples in various programming languages. It also has a blog section where you can learn about the latest updates and features Twilio offers.
How to create a Twilio account
In this section, I will guide you through setting up a Twilio account.
- Go to Twilio homepage and create an account. You will have to verify your email and phone number.
- Head over to your Twilio Console once you’re signed in.
- Generate a Twilio phone number on the dashboard. This phone number is a virtual number that allows you to communicate via Twilio.
- Copy the Twilio phone number somewhere on your computer; to be used later in the tutorial.
- Scroll down to the Account Info section, copy and paste the Account SID and Auth Token somewhere on your computer; to be used later in this tutorial.
Once you have your Twilio phone number, Account SID and Auth Token, we can start coding the coupon generator.
Setting up a Node.js server
In this section, you will create a Node.js server and install Twilio and other necessary packages to the server.
Download and install Node.js from its website; if you do not have Node.js installed on your computer. Then create, a folder that will contain the app.
1mkdir <app-name>
Open your terminal and navigate into the folder.
1cd <app–name>
Create a package.json
file by running the code below. Fill in all the required information.
1npm init
Create an index.js file – the entry point to the web application.
1touch index.js
Install Twilio, Dotenv and Express. With Dotenv, you can load private data such as API keys and passwords into a Node.js application. Express.js is a fast, minimalist framework that provides several features for building web applications in Node.js.
1npm install twilio express dotenv --save
Copy the following code into the index.js file.
1const express = require('express'); //import expressjs
2const app = express();
3const PORT = 4000; // where the server runs
4
5//creates a route that allows only GET request
6app.get('/', (req, res) => {
7 res.send('Hello world!');
8});
9
10//listens to updates made on the project and shows the status of the project
11app.listen(PORT, () => {
12 console.log(`⚡️[server]: Server is running at https://localhost:${PORT}`);
13});
From the code snippet above, I imported Express.js, then declared a specific port where the server runs. app.get()
accepts a route as a parameter, and the forward slash represents the home page. When you visit localhost:4000
in your web browser, “Hello world!” is shown on the screen.
Run the code below in your terminal to start the Node.js server.
1node index.js
Creating the web client using Express.js
Here, we will create a web page containing a text field and a button that allows users to submit the phone number which receives the coupon code.
To create the web client, you will have to:
Update the index.js file to render HTML pages.
1//--> Required imports
2
3const express = require('express');
4const PORT = process.env.PORT || 4000;
5const app = express();
6const path = require('path');
7
8//--> Routes
9app.get('/', (req, res) => {
10 res.sendFile(path.join(__dirname + '/index.html'));
11});
12
13// --> Server listener
14app.listen(PORT, () => {
15 console.log(`⚡️[server]: Server is running at https://localhost:${PORT}`);
16});
Create the index.html page by running the code below.
1touch index.html
Then, update the index.html
file to display a form field that accepts the user’s phone number and a submit button. The form below submits the user’s phone number to the “/sent” route.
1<!DOCTYPE html>
2<html lang="en">
3<head>
4<meta charset="UTF-8" />
5<meta http-equiv="X-UA-Compatible" content="IE=edge" />
6<meta name="viewport" content="width=device-width, initial-scale=1.0" />
7<title>Coupon Generator</title>
8<style>
9* {
10 margin: 0;
11 padding: 0;
12}
13body {
14 min-height: 100vh;
15 width: 100%;
16 background-color: #f2ebe9;
17 display: flex;
18 align-items: center;
19 justify-content: center;
20 flex-direction: column;
21}
22
23form {
24 display: flex;
25 align-items: center;
26 justify-content: center;
27 flex-direction: column;
28 width: 100%;
29}
30
31h2 {
32 margin-bottom: 30px;
33}
34
35input {
36 padding: 15px 20px;
37 border-radius: 5px;
38 outline: none;
39 width: 60%;
40 margin: 20px 0;
41}
42
43button {
44 height: 45px;
45 width: 200px;
46 padding: 10px 0;
47 outline: none;
48 border: none;
49 cursor: pointer;
50 color: aliceblue;
51 background-color: rgb(13, 13, 90);
52}
53</style>
54</head>
55
56<body>
57 <h2>Receive coupons</h2>
58 <form method="POST" action="/sent">
59 <label for="number">
60 Enter your phone number - {+ country code and number}
61 </label>
62 <input type="tel" id="number" placeholder="+234-998975216" name="number"/>
63 <button>SUBMIT</button>
64 </form>
65</body>
66</html>
Add a “/sent” route to the index.js file as done below. This route will accept the user’s phone number and send the coupon to the phone number.
1app.post('/sent', (req, res) => {
2 res.send('Your coupon has been sent to your number!');
3});
Next, run the server to make sure there are no errors.
1node index.js
Connecting the web client to Twilio
Here, you will learn how to get users’ phone numbers from a web page and send SMS to their phones using Twilio.
To access the user’s phone number on the backend server, the body-parser configuration is required. This configuration allows you to access inputs from a form field.
1//--> index.js
2//Should be placed above the app.get() and app.post() routes
3app.use(express.urlencoded({ extended: true }));
Edit the app.post("/sent")
and try logging the user’s number to the terminal.
1app.post('/sent', (req, res) => {
2 const { number } = req.body;
3 console.log(number);
4 res.send('Your coupon has been sent to your number!');
5});
Next, I will guide you through creating the coupon and sending it to the user’s phone number.
First of all, create a function that generates a random set of strings – the coupon code.
1const fetchCoupon = () => {
2 const alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
3 const year = new Date().getFullYear().toString();
4 let coupon = [];
5 for (let i = 0; i < 6; i++) {
6 let index = Math.floor(Math.random() * alphabets.length);
7 coupon.push(alphabets.charAt(index));
8 }
9 return coupon.join('') + year;
10};
From the code snippet above, the alphabet variable is a string containing all the letters in the English alphabet. The year variable gets the current year and converts it to a string. The loop fetches a random set containing six letters and adds them to the coupon variable. All the random letters in the coupon array are converted to strings, concatenated then returned together with the current year as a single string.
Create a .env
file containing the Twilio configuration keys.
1TWILIO_ACCOUNT_SID=<account_SID>
2TWILIO_AUTH_TOKEN=<auth_token>
3TWILIO_PHONE_NUMBER=<twilio_phone_number>
Import the configuration keys into the index.js
file via Dotenv.
1require('dotenv').config(); //imports dotenv
2const accountSid = process.env.TWILIO_ACCOUNT_SID;
3const authToken = process.env.TWILIO_AUTH_TOKEN;
4const myTwilioNumber = process.env.TWILIO_PHONE_NUMBER;
5const client = require('twilio')(accountSid, authToken);
The dotenv package enables us to access data stored in environment variables, which is required for sending messages via Twilio.
Update the app.post("/sent")
route to the code below.
1app.post('/sent', (req, res) => {
2 const { number } = req.body;
3 client.messages.create({
4 body: fetchCoupon(),
5 from: myTwilioNumber,
6 to: number
7}).then((message) => console.log(message, sid));
8 res.send('Your coupon has been sent to your number!');
9});
client.messages.create()
accepts an object containing three keys – body, from, and to. The body key contains the content of the message, a string value returned from function fetchCoupon
. The to
and from
attributes represent the sender and the recipient.
Congratulations! you’ve just completed this project.
Conclusion
In this tutorial, you’ve learnt how to generate a set of random strings that is relevant to different use cases, such as generating API keys for your products and also how to send SMS via Twilio.
Twilio enables you to add various forms of communication to software applications effortlessly. It has helped several organizations, including Stripe, Lyft, and Airbnb, establish excellent customer relationships with their users.
If you need a tool that supports multiple forms of communication with your users using SMS, voice, email, video, or WhatsApp, Twilio is an excellent choice.
You can check the full source code here:
https://github.com/novuhq/blog/tree/main/sending%20SMS%20via%20Twilio
Novu
Novu is the first open-source notification infrastructure for developers. If you want to leverge the power of Twilio with an entire notification infrastructure, check out our library at: https://github.com/novuhq/novu
Thank you for reading!