How to use Handlebars to send emails via SendGrid in a Node.js application
The ability to send email to users is one feature most modern web applications have. Whether you are sending a passcode to the user’s email address before they gain access to a resource or sending a confirmation email or newsletters to users, we tend to need this feature at some point when building web applications.
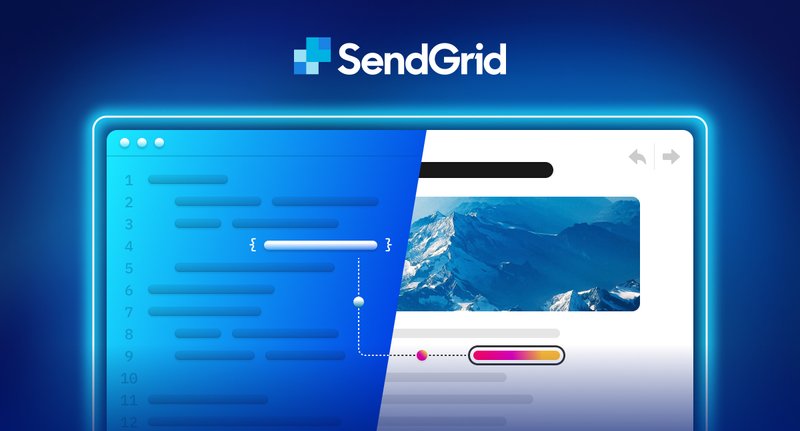
The ability to send email to users is one feature most modern web applications have. Whether you are sending a passcode to the user’s email address before they gain access to a resource or sending a confirmation email or newsletters to users, we tend to need this feature at some point when building web applications.
But without the right tool, sending emails to users can be a challenge which is one of the reasons SendGrid exists. SendGrid enables developers to add different email communication features to web applications painlessly within the shortest time possible.
SendGrid is a fast and scalable service that processes billions of emails monthly. With SendGrid, you can monitor every email you send via the dashboard provided. It is simple and easy to use.
In this tutorial, I will walk you through creating email templates using Handlebars and sending emails via SendGrid. We will first create an HTML template using Handlebars, and then connect the template to a Node.js server where we send the emails using SendGrid.
What is Handlebars?
Handlebars is a JavaScript templating engine that enables you to generate dynamic HTML pages effortlessly. Templating engines like Handlebars convert JavaScript functions into HTML templates and replace variables with actual data.
Node.js has several templating engines, such as EJS, Pug, Nunjucks, and doT but Handlebars stands out because it has a faster execution time and provides a simple syntax for adding dynamic content to your webpage using different layouts.
Why use Handlebars?
- Simple to use
Handlebars have a simple syntax making it easy for new users to understand. You do not need to run many configurations to use Handlebars in your web applications. Once you install it, you can start using the templating engine immediately. - Excellent documentation
Handlebars have excellent documentation, making it easy for new and existing users to navigate and learn about its features rapidly.
Handlebars documentation is short and developer-friendly, and you are less likely to run into bugs because it has detailed documentation. - Ability to create custom helpers
Helpers are JavaScript functions registered by Handlebars to render different templates or HTML layouts. Handlebars allow you to extend the helpers library by creating reusable JavaScript functions for commonly used web layouts. - Faster execution time
Compared to templating engines like Mustache, Handlebars has a faster execution time because it compiles the JavaScript functions into HTML templates once and then calls the compiled function for subsequent usage. - Good architectural design
Handlebars is a simple templating engine with a structured layout making it easy for anyone to read and understand the codebase. Handlebars separate the views and logic into different folders, thus making it a logic-less templating engine.
Next, let’s create an email template using Handlebars on a Node.js server.
Create a Handlebars template in Node.js
- Download and install Node.js from its website, if you don’t have Node.js installed on your computer.
- Create a folder that will contain our app.
- Open your terminal and navigate into the folder.
1cd <app–name>
- Create a package.json file by running the code below. Fill in all the required information.
1npm init
- Create an index.js file – the entry point to the web application.
1touch index.js
- Install Handlebars by running the code below.
1npm install handlebars
- Create a templates folder containing the index.handlebars file.
1mkdir templates
2cd templates
3touch index.handlebars
- Copy the following code into the index.handlebars file
1<!DOCTYPE html>
2<html lang="en">
3<head>
4
5 <meta http-equiv="X-UA-Compatible" content="IE=edge">
6 <meta name="viewport" content="width=device-width, initial-scale=1.0">
7 <title>Message</title>
8</head>
9<body>
10 <p>Hello {{name}}</p>
11 <p>We just received your application and we are pleased to inform you that you are invited to the next stage of interview</p>
12 <p>Your interviewer is {{interviewer}}</p>
13 <p>Best regards,</p>
14 <p>Sheggs Company</p>
15</body>
16</html>
The code snippet above is a Handlebars template that displays the content within the body tag.
Name and interviewer represent variables that store dynamic content retrieved from the compiled template.
Next, let’s learn how to replace these variables with exact values
- Open the index.js file and import Handlebars.
1//in index.js
2const handlebars = require(“handlebars”)
- Import the file system module from Node.js. The file system module allows you to read and write files on your computer system.
1const fs = require(“fs”)
- Create a reference to the Handlebars template. fs.readFileSync() enables us to read a file and return its content.
1const emailTemplate = fs.readFileSync(path.join(__dirname, "/templates/index.handlebars"), "utf-8")
- Install path from Node.js. The path.join() method above creates an absolute URL to the file.
1const path = require(“path”)
- Compile the Handlebars template, and provide the values for the name and interviewer variables.
1const template = handlebars.compile(emailTemplate)
2
3const messageBody = (template({
4name: "David Islo",
5interviewer: "Scott Greenwich"
6}))
- Try logging the messageBody variable to the console, you should retrieve the template’s HTML content.
1console.log(messageBody)
Congratulations, the email template is completed. Next, let’s learn how we can send this template as an email.
How to send Emails using SendGrid
In this section, you will learn how to send emails using SendGrid.
Setting up SendGrid
- Install SendGrid and Dotenv. Dotenv enables us to load data from environment variables into the Node.js web application. The environment variables store private keys, API keys, and passwords.
1npm install dotenv @sendgrid/mail
- Create an empty .env file.
1touch .env
- Visit SendGrid homepage to create an account.
- Enable Two-factor authentication.
- Select API Keys under Settings on the sidebar.
- Create your API Key by selecting Mail Send under Restricted Access.
- Copy and paste your API Key into the .env file.
1SENDGRID_API_KEY=<SG.your_api_key>
- Adding SendGrid to a Node.js server
1// Import SendGrid and Dotenv in the index.js file.
2
3const sgMail = require("@sendgrid/mail")
4require("dotenv").config()
Set SendGrid API key to that of the environment variable.
1sgMail.setApiKey(process.env.SENDGRID_API_KEY)
Copy the code below
1const messageInfo = {
2 to: "<email_recipient>",
3 from: "<registered_sendgrid_email>",
4 subject: "Congratulations! You are made it!",
5 html: messageBody //Handlebars template
6}
7
8//Pass the messageInfo object into SenGrid send function
9sgMail.send(messageInfo, (error, result) => {
10 error ? console.log("Email not sent!") : console.log("Email Sent!")
11})
From the code snippet above:
- I created a messageInfo object that accepts all the needed email parameters from the function. The
html
key enables us to send HTML elements as a message to the recipient. - The sgMail.send() function sends the email to the recipient and returns a callback function if there is an error.
Conclusion
In this tutorial, you have learned how to create email templates using Handlebars and send emails using SendGrid in a Node.js application.
SendGrid makes it easy to integrate email communication into web applications at zero cost. Whether you are sending a single text or using a templating engine such as Handlebars, SendGrid can handle both tasks efficiently.
If you want to build a web application that enables you or users to send and receive emails, SendGrid is an excellent choice.
Thank you for reading!