Send Whatsapp Notifications with Next.js and Novu
How to send Whatsapp Notifications with Next.js and Novu
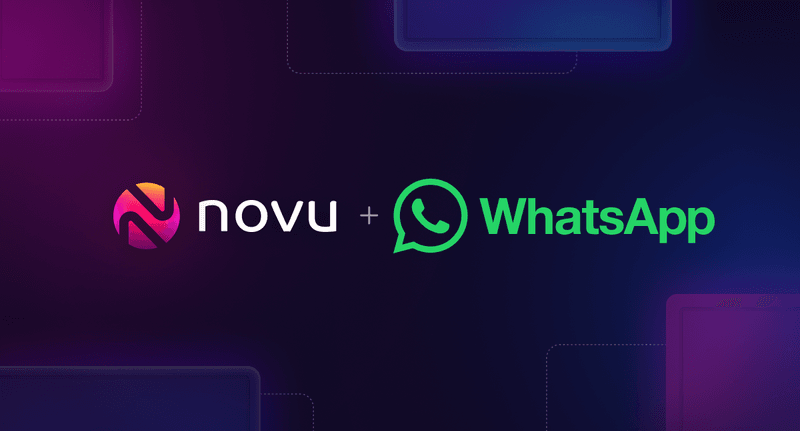
As a business, offering the option to send transactional notifications to our users’/clients’ WhatsApp chat is a must-have. Use cases and business needs change, but the foundation is usually very similar across the majority.
As developers, we know that WhatsApp is only ONE OF the potentially required channels of communication, and being smart means not sweating too much on building staff and infrastructure, someone has already built and shared with the world. Check out Novu.
This article assumes you know what a notification workflow is and why you should use it. It also assumes you are aware of Next.js and WhatsApp chat app.
Novu recently released a new way of baking notification workflows into your apps via code.
To learn about it, visit the related documentation. You might also benefit from reading the code-first approach to managing notification workflows article.
If you prefer to get your hands dirty quickly, go ahead and clone the code repo from GitHub.
1. Initialize a new NEXT.JS Project
We will follow the [official installation guide from NEXT](https://nextjs.org/docs/getting-started/installation), open your terminal / IDE, and run the following command:
1npx create-next-app@latest
2
On installation, you’ll see the following prompts:
1What is your project named? my-app
2
3Would you like to use TypeScript? No / Yes
4
5Would you like to use ESLint? No / Yes
6
7Would you like to use Tailwind CSS? No / Yes
8
9Would you like to use `src/` directory? No / Yes
10
11Would you like to use App Router? (recommended) No / Yes
12
13Would you like to customize the default import alias (@/*)? No / Yes
14
15What import alias would you like configured? @/*
We will not use `/src` directory in this guide.
2. Integrate Novu Echo Into Our App (Notification Workflow)
1. We will install the `@novu/echo` package in the root of our NEXT app directory by running the following command:
1npm install @novu/echo
2. We must provide an API endpoint for the Novu Dev Studio to fetch our notification workflow. (Don’t worry; we’ll guide you through all the steps.) Navigate to your app’s `app/` directory and create a new directory named `api`. Within the `api` directory, we will create one additional directory and name it `echo`.
1cd app
2
3mkdir api
4
5cd api
6
7mkdit echo
Create a file within the `app/api/echo` directory and name it `route.ts`. Copy and paste the code snippet below:
1../app/api/echo/route.ts
2
3import { serve } from "@novu/echo/next";
4
5import { echo } from "../../echo/novu-workflow";
6
7export const { GET, POST, PUT } = serve({ client: echo });
3. Now, let’s create the instance where we will build and maintain our notification workflow:
Navigate to your `app` directory and create another directory named `echo`.
1cd app
2
3mkdir echo
Create a file within the `app/echo` directory and name it `novu-workflow.ts`. Copy and paste the code snippet below:
1// ..app/echo/novu-workflow.ts
2
3import { Echo } from "@novu/echo";
4
5export const echo = new Echo({
6 /**
7 * Enable this flag only during local development
8 */
9 devModeBypassAuthentication: process.env.NODE_ENV === "development",
10});
11
12echo.workflow(
13 "hello-world",
14 async ({ step }) => {
15 await step.chat(
16 "send-chat",
17 async () => {
18 return {
19 body: "Hello from Novu",
20 };
21 },
22 {
23 inputSchema: { // 👈 This is the input schema for the current step
24 type: "object",
25 properties: {},
26 },
27 },
28 );
29 },
30 {
31 payloadSchema: { // 👈 This is the payload schema for the whole workflow
32 type: "object",
33 properties: {}
34 }
35 },
36);
In the `body` property in the code above, we can define what kind of notification (or message) the user/client will get to one’s WhatsApp client app.
We haven’t finished the article yet but have everything to build a notification workflow.
Below, we can see a bare-bone Novu echo instance to shape your desired workflow.
1//..app/server/echo/novu-workflow.ts
2// This is Echo Client instance concept - not a working instance!
3
4import { Echo } from '@novu/echo';
5
6export const echo = new Echo({
7 /**
8 * Enable this flag only during local development
9 * For production this should be false
10 */
11 devModeBypassAuthentication: true
12});
13
14echo.workflow(
15 '<WORKFLOW_NAME>', // The Workflow name. Must be unique across your Echo client.
16 // Workflow resolver, the entry-point for your Workflow steps
17 async ({
18 // Helper function to declare your Workflow Steps.
19 step,
20 // Workflow Trigger payload
21 payload,
22 }) => {
23 // ...your Workflow Steps
24}, {
25 // JSON Schema for validation and type-safety. Zod, and others coming soon.
26 // <https://json-schema.org/draft-07/json-schema-release-notes>
27
28 // The schema for the Workflow payload passed dynamically via Novu Trigger API
29 // Defaults to an empty schema.
30 payloadSchema: { properties: { name: { type: 'string' }}},
31 // The schema for the Workflow inputs passed statically via Novu Web
32 // Defaults to an empty schema.
33 inputSchema: { properties: { brandColor: { type: 'string' }}},
34});
We can:
– Select a name for our workflow.
– Declare Workflow Steps as we see fit for our use case. It can be a single-step or multi-step workflow
– Configure what could be passed in the payload of the workflow trigger [payloadSchema].
– Configure what inputs could be passed statically via Novu Web ([inputSchema](https://docs.novu.co/echo/concepts/inputs))
Note: You can create and manage as many workflows as you wish within `app/api/echo/novu-workflow.ts`; make sure to provide each workflow with a unique name.
—
3. Adding WhatsApp Business as a provider to Novu Integrations Store
To integrate WhatsApp Business with Novu, you must create a Facebook developer app and obtain the necessary credentials.
Step 1: Create a Facebook Developer App
Visit the Facebook Developer Portal and create a new app.
Select “Other” for “What do you want your app to do?” and select “Business” for “Select an app type”.
Step 2: Setup WhatsApp Product
On the App Setup page, click on “Set Up” under the “WhatsApp” product. You must create or add a Facebook Business Account to your app.
Step 3: Send a Sandbox Message
Copy the following pieces and paste them in the Novu WhatsApp Business integration settings:
– Temporary Access Token – Access API token Field
– Phone Number ID – Phone Number Identification Field
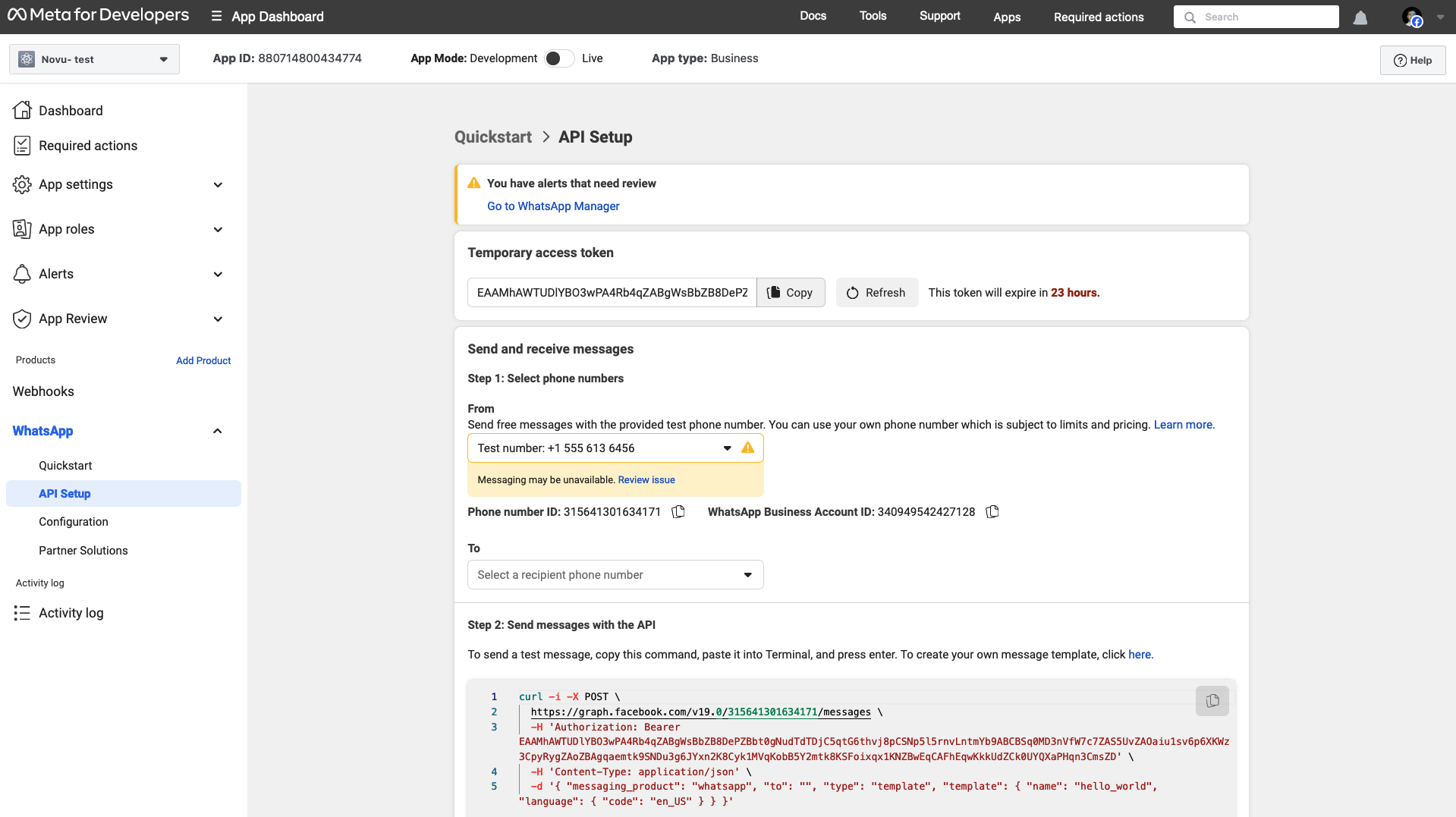
Note: It’s important to note that the test Whatsapp credentials cannot be used in production and will expire in 24 hours.
You will need to submit your app for review to obtain production credentials.
Step 4: Add a Test Phone Number
You can add a test phone number to the sandbox by clicking on the “Add Phone Number” button.
This number can be used to test your integration with Novu before submitting it for review.
Step 5: Creating a WhatsApp Template (Optional / For Production)
For test credentials, we can only use pre-approved templates. So you can skip this step if you don’t want to wait for the approval of your template and mainly test the functionality.
Here is an example of how a notification is sent to the `RECIPIENT` by passing the approved template as a `template.name` property and calling Meta’s API endpoint:
1curl -i -X POST \
2
3https://graph.facebook.com/v19.0/<PHONE_NUMBER_ID>/messages \
4
5-H 'Authorization: Bearer <ACCESS_TOKEN>' \
6
7-H 'Content-Type: application/json' \
8
9-d '{ "messaging_product": "whatsapp",
10
11"to": "<RECIPIENT_PHONE_NUMBER>",
12
13"type": "template",
14
15"template": {
16
17"name": "hello_world", //Varified or Approved template
18
19"language": {
20
21"code": "en_US" }
22
23}
24
25}'
Learn more about how to create and manage WhatsApp templates.
5.1 Register a Business Phone Number
To go live you will need to add a real business phone number and submit your app for review.
Follow the Facebook Instructions on how to proceed.
5.2 Generate a permanent access token
Follow the Facebook Instructions on how to generate a permanent access token.
Depending on your use case.
5.3 Creating a WhatsApp Template
We must create a WhatsApp Template to send notifications to your customers. Create a template in the Business Manager and submit it for review.
After your template is approved, we can use the `template_name` to send notifications to your customers.
—
4. Launching The Dev Studio
Now that everything in our notification workflow is configured and the WhatsApp provider is already integrated into the Novu Integration Store, it’s time to see a visual representation of what we have built.
1. If you haven’t run the development environment for your NEXT app, now is the time.
1npm run dev
2. Do you remember that we exposed an echo API endpoint in our app for Dev Studio to catch? This is where it happens.
Run the following command in a separate terminal:
1npx novu-labs@latest echo
If you’ve followed this post, you should see this:
Here is where our exact API endpoint goes. If our application runs on a different port, we should change the URL manually to point Dev Studio in the right direction.
Also, if we have configured everything correctly, we should see that Dev Studio sees our workflow identifier (Workflow unique name).
3. We press the “View Workflow” button in the Dev Studio to see our workflow.
We should see the following:
4. Press on the workflow step node called “send-chat.” We should see a preview of our chat body and the `Step Input` and `Payload` variables we have configured in the workflow schemas.
5. Feel free to adjust the chat text, step input schema, or define the properties we anticipate in the payload. This UI shows a live representation of everything. You can add more steps like In-App, SMS, and Email in code and it will be shown visually in the Dev Studio.
—
5. Syncing our workflow to Novu Cloud
Having completed crafting, designing, and modifying our notification workflow to suit our requirements, we’re ready to push it to Novu Cloud. There, it will handle the heavy lifting and seamlessly execute all the steps we’ve configured whenever we trigger the workflow.
1. Click on the “Sync to Cloud” button on the top right.
2. We will need to create a local tunnel that the Novu Cloud environment can reach for local experimentation purposes.
On a separate terminal, run the following command:
1// Change to the port where the app is currently running
2
3npx localtunnel --port 3000
We should get something like:
1your url is: <https://famous-pumas-clap.loca.lt>
3. Click on the “Create Diff” button. To push (merge) the workflow code to the cloud, an API Key from our Novu account should be added to our echo instance.
Let’s navigate to `../app/echo/novu-workflow.ts` file and add our Novu API Key.
1// ..app/echo/novu-workflow.ts
2
3import { Echo } from "@novu/echo";
4
5export const echo = new Echo({
6 apiKey: process.env.NOVU_API_KEY, // <<-- Your Novu API KEY
7 /**
8 * Enable this flag only during local development
9 */
10 devModeBypassAuthentication: process.env.NODE_ENV === "development",
11});
12
13echo.workflow(
14 "hello-world",
15 async ({ step }) => {
16 await step.chat(
17 "send-chat",
18 async () => {
19 return {
20 body: "Hello from Novu",
21 };
22 },
23 {
24 inputSchema: { // 👈 This is the input schema for the current step
25 type: "object",
26 properties: {},
27 },
28 },
29 );
30 },
31 {
32 payloadSchema: { // 👈 This is the payload schema for the whole workflow
33 type: "object",
34 properties: {}
35 }
36 },
37);
We will now click the “Create Diff” button again.
This is where you can review all the changes made to the workflow. Once you have verified that everything is in order, click the “Deploy Changes” button.
—
6. Testing Our Notification Workflow
There are many ways to test and trigger our workflow, but we’ll make a `cURL` API call to Novu Cloud from our terminal in this article.
If we don’t have any subscribers or users in our database or within our Novu Cloud organization, we’ll send the test to ourselves for simplicity.
– In the `subscriberId` key, input a random number or even our email address (as long as we do not try to assign the same ID key to another subscriber, we should be good).
– For the `phone` key, we will need to insert a valid phone number so we can actually receive the message.
Note: Learn more about the structure of subscriber properties.
We can leave the payload empty or add properties aligned with the `payloadSchema` we established in the echo notification workflow instance.
1
2curl -X POST <https://api.novu.co/v1/events/trigger> \\
3 -H "Authorization: ApiKey <NOVU_API_KEY>" \\
4 -H "Content-Type: application/json" \\
5 -d '{
6 "name": "hello-world",
7 "to": {
8 "subscriberId": "<UNIQUE_SUBSCRIBER_IDENTIFIER>",
9 "phone": "<VALID_PHONE_NUMBER>",
10 },
11 "payload": {}.
12 "overrides": {
13 "chat": {
14 "template": {
15 "name": "template_name",
16 "language": {
17 "code": "en_US"
18 }
19 }
20 }
21 }
22 }
23}'
This is how it’s going to look like in Novu Cloud workflow editor.
Once we run the trigger the workflow, we will get some sort of indication about the execution.
If we have done everything correctly, we should get a Whatsapp message.
Additional resources:
– How to send WhatsApp Business notifications with Novu
– Meta For Developers: Template Messages
– Sample message templates for your WhatsApp Business account